Data Item
- A data item is a name used to hold the value for processing in the program. It is also called as a Variable.
- The variable declaration should always contain the data type and its length (except the group variable).
- Based on the size of the data, it is a pointer to the allocated memory location from the starting to the ending byte.
- All variables should be declared in the DATA DIVISION.
Syntax -
level-number variable-name
[PIC/PICTURE data-type-character(variable-length)]
[VALUE literal-value].
For Example - Declaring a variable of numeric type to store a value 123.
01 WS-NUMERIC-VAR PIC 9(03) VALUE 123.
- level-number - Refers to the level number of the declaration from 01 to 49. From the example, it is 01.
- variable-name - Refers to the name of the variable. From the example, it is WS-NUMERIC-VAR.
- data-type-character - Refers to the type of the variable. From the example, it is numeric(9).
- variable-length - Refers to the variable length to store the data. From the example, it is 03.
- literal-value - Refers to the initial value assigned to the variable. From the example, it is 123.
Variable Naming Rules -
- The variable name is a combination of COBOL user-defined words that are formed by alphabets(A-Z), digits(0-9) and hyphens (-).
- Variable name length can be a minimum of 1 character and a maximum of 30 characters.
- Variable name should not be a COBOL reserved word(i.e., ACCEPT, ADD, MOVE, etc..).
- The variable name does not allow spaces between the words and should combine them with '-'. Starting or ending character should be an alphabet(A-Z) or a digit(0-9) but not a hyphen(-) or space.
For example - Valid and Invalid variable names
Valid | Invalid |
---|---|
WS-A VAR A12 1A2 WS-2 WS WS |
WS_A WS* MOVE WS-$ WS A -WS WS- |
Variable types -
The variables are divided into three types based on their declaration and those are -
- Individual variable
- Group variable
- Elementary variable
Individual variable -
- An individual variable is declared individually but neither under a group variable nor as a group variable.
- It always has the picture clause during its declaration.
- It should be unique in the program or its calling programs.
- It can be declared using level numbers 01 to 49 and 77.
For example - Declaring individual variable.
----+----1----+----2----+----3----+----4----+----5----+
* INDIVIDUAL VARIABLE
01 LEVEL-1 PIC 9(03) VALUE 256.
Group variable -
- A Group variable is declared without the picture clause and has elementary variables declared under it.
- It does not have a picture clause.
- Its name should be unique in a program or its calling programs.
- These can be declared using level numbers from 01 to 48.
For example - Declaring a group variable with two elementary variables.
----+----1----+----2----+----3----+----4----+----5----+
* Group Variable
01 LEVEL-GROUP.
* Elementary Variables
05 LEVEL-21 PIC 9(03) VALUE 256.
05 LEVEL-22 PIC 9(03) VALUE 128.
Elementary variable -
- The elementary variable is a variable that is declared under the group variable.
- It should always have a PIC clause.
- Elementary variables are not unique and can use the same name under different group variables. In this case, the elementary variables use the OF keyword followed by the group variable name.
- It can be declared using level numbers from 02 to 49.
For example - Declaring a group variable with two elementary variables.
----+----1----+----2----+----3----+----4----+----5----+
01 LEVEL-GROUP.
* ELEMENTARY VARIABLE
05 LEVEL-21 PIC 9(03) VALUE 256.
05 LEVEL-22 PIC 9(03) VALUE 128.
Memory allocation understanding example -
Let's take the example below to understand it better. A, B & C are variables and are declared as shown below -
01 A PIC X(10).
01 B.
02 C PIC X(03).
02 FILLER PIC X(03).
Let us assume variable A allocated 10 bytes of memory from 1000 to 1010 and variable B with 6 bytes from 1100 to 1106. The below diagram represents memory allocation for the declaration -
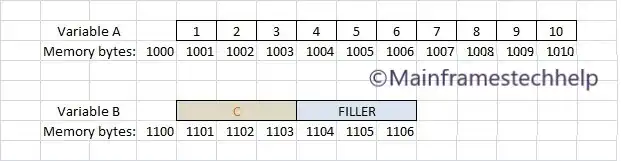
Variable A is allocated with the memory location from 1000 to 1010. So, referring to the variable A, it accesses the 10-byte data from memory location 1000 to 1010. Variable C is allocated with the memory location from 1100 to 1103. So, the variable C accesses the 3-byte data from memory location 1100 to 1103.
We can't access the FILLER directly because it is not a variable. However, variable B is declared as a group variable for 6 bytes, including variable C and FILLER. So, by referring to variable B, we can access the 6 bytes of data from memory.
Practical Example -
Scenario - Different variable types declaration, initialization and display in COBOL program.
Code -
----+----1----+----2----+----3----+----4----+----5----+
...
WORKING-STORAGE SECTION.
* Individual variable
01 LEVEL-1 PIC 9(03) VALUE 256.
* Group variable
01 LEVEL-GROUP.
* Elementary variables
05 LEVEL-21 PIC 9(03) VALUE 256.
05 LEVEL-22 PIC 9(03) VALUE 128.
...
PROCEDURE DIVISION.
DISPLAY 'INDIVIDUAL VARIABLE : ' LEVEL-1.
DISPLAY 'GROUP VARIABLE : ' LEVEL-GROUP.
DISPLAY 'ELEMENTARY VARIABLE-1 : ' LEVEL-21.
DISPLAY 'ELEMENTARY VARIABLE-2 : ' LEVEL-22.
...
Output -
INDIVIDUAL VARIABLE : 256 GROUP VARIABLE : 256128 ELEMENTARY VARIABLE-1 : 256 ELEMENTARY VARIABLE-2 : 128