COBOL OCCURS Clause
- OCCURS clause is used to define arrays (or tables).
- It allows a data item to be repeated a specified number of times.
- This is particularly useful for handling collections of similar items, such as a list of employee names or a series of monthly totals.
Syntax -
OCCURS integer1 [TO integer2] TIMES
[DEPENDING ON data-name]
[DESCENDING|ASCENDING KEY IS key-var]
[INDEXED BY index-name]
...
- integer1 - The number of times the data item should be repeated.
- integer1 [TO integer2] - integer1 is minimum number of times and integer2 to maximum number of times. TO is applicable when DEPENDING ON is coded.
- DEPENDING ON data-name - This makes the table variable-length, with its size determined by the current value of data-name.
- DESCENDING|ASCENDING KEY IS key-var - Specifies the array sorting order using key-var.
- INDEXED BY index-name - This defines an index for the table. The index can be used to reference specific occurrences within the table.
How the data stored in memory for table OCCURS?
Mostly, the repeated data items generally store in continuous memory locations. Let’s use the single dimensional array example and assume that it uses the continuous memory to store the data. The declaration as follows -
01 WS-CLASS.
03 WS-STUDENT OCCURS 2 TIMES.
05 WS-ROLL-NO PIC X(03) VALUE "001".
05 WS-NAME PIC X(10) VALUE "PAWAN Y".
The below diagram shows the memory storage, if they are stored in subsequent memory locations.
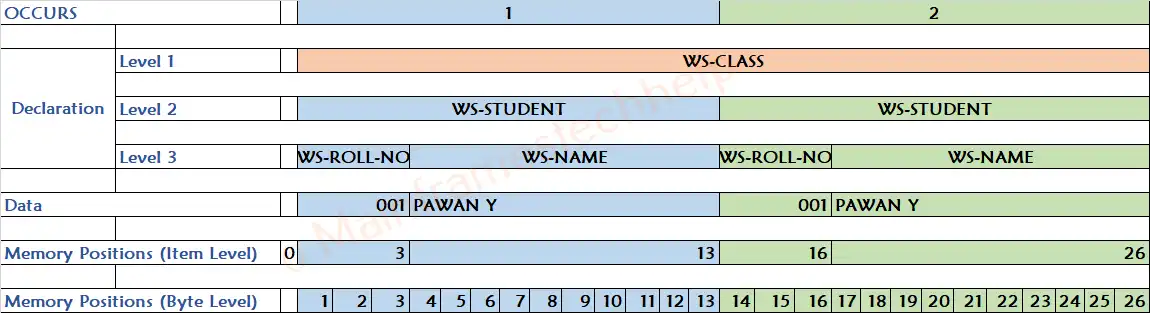
In the above diagram, OCCURS represent the how many times the data repeated. A memory positions (Item Level) represents the OCCURRENCE level memory representation because each occurrence occupies 13 bytes according to the declaration. Memory positions (Byte level) represent each byte level memory representation.
How many types of tables?
COBOL supports two types of tables based on declaration –
Fixed-length tables -
The number of OCCURS has a static value. If any changes needed to the number of OCCURS that requires a program modification.
Scenario - Declare a table to store 10 student information.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-CLASS.
05 WS-STUDENT OCCURS 10 TIMES.
10 STUDENT-ID PIC 9(5).
10 STUDENT-NAME PIC X(30).
Explaining Example -
In this example:
- WS-CLASS is a table that has 10 occurrences of WS-STUDENT.
- Each WS-STUDENT consists of a STUDENT-ID (numeric) and a STUDENT-NAME (alphanumeric).
- The table is of fixed length, i.e., it always has space for 10 student's details. We can't add an 11th student without changing the program and recompiling.
Variable-length tables -
The number of OCCURS value will be decided by using variable at the runtime of the program.
Scenario - Declare a table to store student information and the number of students is not known currently.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-STUDENT-CNT PIC 9(03).
01 WS-CLASS.
05 WS-STUDENT OCCURS 10 TIMES
DEPENDING ON WS-STUDENT-CNT.
10 STUDENT-ID PIC 9(5).
10 STUDENT-NAME PIC X(30).
PROCEDURE DIVISION.
ACCEPT WS-STUDENT-CNT.
Explaining Example -
In this example:
- WS-CLASS is a table that has max 10 occurrences. However, the actual value gets decided by WS-STUDENT-CNT variable.
- Each WS-STUDENT consists of a STUDENT-ID (numeric) and a STUDENT-NAME (alphanumeric).
- The table is of variable length, i.e., its length always decided by the value of WS-STUDENT-CNT.
Practical Example -
Scenario - Let us declare a table to process two student details. WS-CLASS is the group variable and WS-STUDENT is a variable with all student information OCCURS 2 times to capture the two students information.
Code -
----+----1----+----2----+----3----+----4----+----5----+
...
WORKING-STORAGE SECTION.
* Single Dimensional table
01 WS-CLASS.
03 WS-STUDENT OCCURS 2 TIMES.
05 WS-ROLL-NO PIC X(03) VALUE "001".
05 WS-NAME PIC X(10) VALUE "STUDENT1".
...
PROCEDURE DIVISION.
DISPLAY "CLASS INFO: " WS-CLASS.
...
JCL to execute the above COBOL program −
//MATEPKRJ JOB MSGLEVEL=(1,1),NOTIFY=&SYSUID //* //STEP01 EXEC PGM=SINDIMTB //STEPLIB DD DSN=MATEPK.COBOL.LOADLIB,DISP=SHR //SYSOUT DD SYSOUT=* //SYSIN DD * 03 /*
When the program compiled and executed, it gives the following result with only three occurences −
CLASS INFO: 001STUDENT 001STUDENT 001STUDENT