COBOL Conditional Statements
A conditional statement derives a condition's truth value (TRUE or FALSE), and decides the further program execution flow. These statements allow decision-making in the program based on the evaluation of conditions.
A conditional statement can be divided into either a simple conditional statement (such as IF or EVALUATE) or a conditional statement that consists of an imperative statement that includes a conditional expression.
We can end a conditional statement with either an implicit scope terminator (.) or an explicit scope terminator (END-IF or END-EVALUATE). An explicit terminator converts the conditional statement into a delimited scope terminator (END-IF or END-EVALUATE).
The conditional statements are -
Statement | Description |
---|---|
IF Statement Detailed Tutorial | IF statement validates a condition to get the truth value and executes the statements under IF when the condition is TRUE.
IF statements are of three types based on their usage in the program -
|
EVALUATE Statement Detailed Tutorial | EVALUATE validates multiple conditions at a time and controls the program flow.
It is a shorter form for the nested IF...ELSE and simplifies the logic when multiple choices are available.
EVALUATE logically divided into the below types based on their usage in the program -
|
Conditional Expressions Detailed Tutorial | Conditional expression is a condition or a part of a condition that evaluates either TRUE or FALSE.
These are used to choose the execution flow based on the truth value of the condition. They are -
|
Some statements only work with conditional statements, and those are -
Statement | Description |
---|---|
CONTINUE Detailed Tutorial | CONTINUE statement transfers the control to the immediate COBOL statement that comes next in the program flow. It is a no-operation, and it is a do-nothing statement. |
NEXT SENTENCE Detailed Tutorial | NEXT SENTENCE transfers the control to the following COBOL statement immediately after the explicit scope terminator (period - '.') in the flow. |
Statements with conditional phrases -
If any statement uses the SIZE ERROR, OVERFLOW, AT END, INVALID KEY, AND EXCEPTION phases, those are also called conditional statements. Below COBOL statements become conditional when a condition is coded with them -
Arithmetic Statements -
- ADD...[NOT] ON SIZE ERROR
- SUBTRACT...[NOT] ON SIZE ERROR
- MULTIPLY...[NOT] ON SIZE ERROR
- DIVIDE...[NOT] ON SIZE ERROR
- COMPUTE...[NOT] ON SIZE ERROR
String Handling -
Table Handling -
Input Output Handling -
- READ...[NOT] AT END
- READ...[NOT] INVALID KEY
- START...[NOT] INVALID KEY
- WRITE...[NOT] INVALID KEY
- REWRITE...[NOT] INVALID KEY
- DELETE...[NOT] INVALID KEY
Ordering -
Program or method linkage -
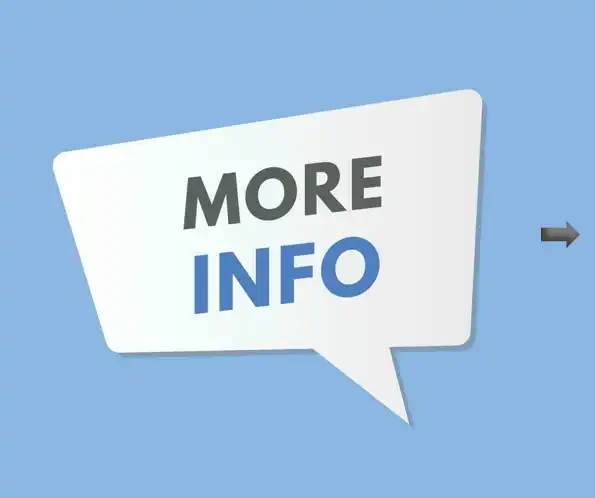