COBOL Data Types
- A data type defines the type of data a variable can hold, such as numeric values, alphabetic characters, or alpha-numeric strings.
- It specifies the nature of the data (e.g., numbers or letters), its format (e.g., decimal places or positions), and the operations can perform on it.
- Data types are always coded with a PICTURE (PIC) clause.
- All variables declarations should be done in DATA DIVISION.
There are five data types in COBOL, and those are -
- Alphabetic
- Alpha-numeric
- Numeric
- Sign
- Decimal Point
Alphabetic Data Type
The alphabetic data type is used to declare the variables for processing the alphabetic strings.
- PICTURE Symbol - A
- Allowed Characters - Space * + - / = $ Comma(,) ; Decimal point(.) " ' ( ) > < : _ A-Z a-z
- Maximum Length - 255
Each character in the string has to be counted to calculate the length, which should be coded after the PICTURE symbol "A". For Example - Declaring a variable to store 2-character string should have the declaration as AA or A(2).
01 WS-ALP PIC A(02).
Numeric Data Type
Numeric data type is used to declare the variables to store and process the numbers or decimal values.
- PICTURE Symbol - 9
- Allowed Characters - 0 to 9
- Maximum Length - 18
Each digit in the number has to be counted to calculate the length, which should be coded after the PICTURE symbol 9. For Example - Declaring a variable to store a 3-digit value should have the declaration as 999 or 9(3).
01 WS-NUM PIC 9(3).
Alpha-numeric Data Type
An alphanumeric data type is used to declare the variables for processing the alpha-numeric strings.
- PICTURE Symbol - X
- Allowed Characters - Space * + - / = $ Comma(,) ; Decimal point(.) " ' ( ) > < : _ A-Z a-z 0-9
- Maximum Length - 255
Each character in the string has to be counted to calculate the length, which should be coded after the PICTURE symbol "X". For Example - Declaring a variable to store a 5-character string should have the declaration as XXXXX or X(5).
01 WS-ALPN PIC X(5).
Sign Data Type
Sign data type is used to declare the numeric variable with the sign to capture the negative values. i.e., sign data type always comes up with numeric data type.
- PICTURE Symbol - S
- Allowed Characters - - (minus) or + (plus)
- Maximum Length - 1 (If SIGN Separate). Otherwise, 0.
Sign value doesn't require any additional byte to store, and it always overpunches on the last digit of the value if the SIGN clause is not coded. For Example - Declaring a variable with sign.
01 WS-SALARY PIC S9(5) VALUE +10000.
Output -
WS-SALARY: 1000{Sign Clause
The SIGN clause is used with SIGN data type to set the sign to be stored in an additional byte. For Example - Declaring two variables with sign separate using SIGN clause. Both occupies 4 bytes.
01 WS-SIGN-LS-P PIC S9(03) VALUE +256
SIGN IS LEADING SEPARATE CHARACTER.
01 WS-SIGN-TS-P PIC S9(03) VALUE +256
SIGN IS TRAILING SEPARATE CHARACTER.
Output -
WS-SIGN-LS-P: +256 WS-SIGN-TS-P: 256+
Decimal point Data Type
When an input is decimal, we should declare a variable with a decimal point to handle it. Decimal point declares only with the combination of numeric data type. The Decimal point declarations are two types -
Real Decimal Point | Dot -
Dot | period (.) is used with the variable declaration to process the decimal value along with dot. The Dot is counted as part of the variable length. For Example - Declaring a variable to store the decimal value 1234.55
01 WS-DECIMAL-VAL PIC 9(4).9(2) VALUE 1234.55.
WS-DECIMAL-VALUE contains 1234.55. When we display WS-DECIMAL-VAL, 1234.55 gets displayed and there is no change in the display.
Assumed Decimal Point | Implied Decimal Point -
"V" is used with a variable declaration to process the decimal value in arithmetic calculations. The "V" is not part of the data and does not count in the variable length. For Example - Declaring a variable with assumed decimal to store the decimal value 1234.55
01 WS-DECIMAL-VAL PIC 9(4)V9(2) VALUE 1234.55.
WS-DECIMAL-VALUE contains 1234.55. When we display WS-DECIMAL-VAL, it displays as 123455, and the decimal point is ignored in the display. However, the value in the WS-DECIMAL-VAL is 1234.55.
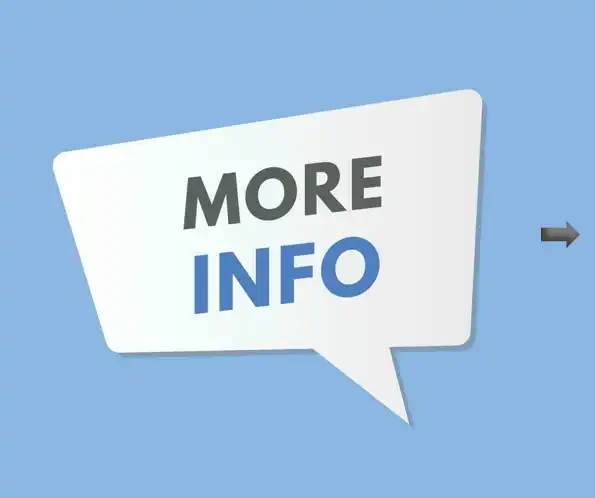