COBOL Table | Array
An array is a collection of individual data items of the same type and length. COBOL arrays are also known as tables. They are a linear data structure that stores identical data repeated multiple times, for example - student marks for all subjects.
Declaration without using table concept to store six subject marks of a student as follows -
01 WS-STUDENT.
03 WS-SUBJECT1-MARKS PIC 9(03).
03 WS-SUBJECT2-MARKS PIC 9(03).
03 WS-SUBJECT3-MARKS PIC 9(03).
03 WS-SUBJECT4-MARKS PIC 9(03).
03 WS-SUBJECT5-MARKS PIC 9(03).
03 WS-SUBJECT6-MARKS PIC 9(03).
The subject marks in the above example have the same type and length. So we can apply the concept of the table by setting the number of subjects the student has, and the above declaration after applying is –
01 WS-STUDENT.
03 WS-SUBJECT-MARKS PIC 9(03) OCCURS 6 TIMES.
Table Declaration –
Tables should be declared in the DATA DIVISION. The OCCURS clause specifies the number representing how many times the data item is repeated in the table.
Syntax -
01 table-name.
02 variable [PIC data-type(length1)]
OCCURS integer1 [TO integer2] TIMES
[DEPENDING ON data-name]
[DESCENDING|ASCENDING KEY IS key-var]
[INDEXED BY index-name]
...
Parameters -
- table-name - Specifies the table name.
- variable - Specifies the repeating element name.
- integer1 - Specifies the number of times the data item should be repeated.
- integer1 [TO integer2] - Specifies integer1 is minimum number of times and integer2 to maximum number of times. TO is applicable when DEPENDING ON is coded.
- DEPENDING ON data-name - Specifies the table as variable-length, and its size is decided at runtime by the value of data-name.
- DESCENDING|ASCENDING KEY IS key-var - Specifies the array sorting order using key-var.
- INDEXED BY index-name - Defines an index for the table. The index can be used to reference all occurrences of the table for processing.
There are two parts in the table. i.e., the first is the table name and the second is the data item (variable).
Rules to Remember -
- The table name is a group variable and should be declared with 01 level. It should not have an OCCURS clause associated with it.
- Data items declared with the OCCURS clause should use the level numbers from 02 to 49.
- OCCURS and PICTURE clauses can be coded together, but not mandatory.
- OCCURS clause should not code with level numbers 01, 66, 77, or 88.
- Each OCCURS is called a dimension, and up to 7 nested dimensions can be coded in a table.
Single Dimensional Table -
One OCCURS clause represents one dimension. We need to code one OCCURS clause to declare a single-dimensional array.
Scenario - Declare a table to save to students information.
Requirement - Let us declare a table to process two student details. WS-CLASS is the group variable and WS-STUDENT is a variable with all student information OCCURS 2 times to capture the two students information.
Code -
----+----1----+----2----+----3----+----4----+----5----+
...
DATA DIVISION.
WORKING-STORAGE SECTION.
* Single Dimensional table
01 WS-CLASS.
03 WS-STUDENT OCCURS 2 TIMES.
05 WS-ROLL-NO PIC X(03) VALUE "001".
05 WS-NAME PIC X(10) VALUE "STUDENT1".
...
PROCEDURE DIVISION.
DISPLAY "CLASS INFO: " WS-CLASS.
...
JCL to execute the above COBOL program −
//MATEPKRJ JOB MSGLEVEL=(1,1),NOTIFY=&SYSUID //* //STEP01 EXEC PGM=SINDIMTB //STEPLIB DD DSN=MATEPK.COBOL.LOADLIB,DISP=SHR //SYSOUT DD SYSOUT=*
When the program compiled and executed, it gives the following result −
CLASS INFO: 001STUDENT1 001STUDENT1
Two Dimensional Table -
An OCCURS clause should be coded within another OCCURS to declare two-dimensional table.
Scenario - Two dimensional table.
Requirement - Let us declare a table to store two student details with 6 subjects marks. WS-STUDENT is variable with all student information OCCURS 2 times to capture the two students information. WS-MARKS-GRP is variable that OCCURS 6 times to capture 6 subjects marks.
Code -
----+----1----+----2----+----3----+----4----+----5----+
...
DATA DIVISION.
WORKING-STORAGE SECTION.
* Two dimensional table
01 WS-CLASS.
03 WS-STUDENT OCCURS 2 TIMES.
05 WS-ROLL-NO PIC X(03) VALUE "001".
05 WS-NAME PIC X(10) VALUE "STUDENT1".
05 WS-MARKS-GRP OCCURS 6 TIMES.
10 WS-MARKS PIC 9(03) VALUE 077.
...
PROCEDURE DIVISION.
DISPLAY "CLASS INFO: " WS-CLASS.
...
JCL to execute the above COBOL program −
//MATEPKRJ JOB MSGLEVEL=(1,1),NOTIFY=&SYSUID //* //STEP01 EXEC PGM=TWNDIMTB //STEPLIB DD DSN=MATEPK.COBOL.LOADLIB,DISP=SHR //SYSOUT DD SYSOUT=*
When the program compiled and executed, it gives the following result −
CLASS INFO: 001STUDENT1 077077077077077077001STUDENT1 077077077077077077
How the table accessed in the program?
There are two ways to access the table in the program –
- Subscript - Subscript is a number of occurrences of a table. We can access table elementary items by using the occurrence number as a subscript.
- Index - The index is the number of displacement positions from the table starting. The system calculates the index value, which we can use to access table elementary items.
We will discuss about these topics in the next immediate chapters.
How many types of tables?
COBOL supports two types of tables based on declaration –
- Fixed-length tables - Specify the number of OCCURS as a static value. Any changes in the number of OCCURS require a change in the program code.
- Variable-length tables - Specify the number of OCCURS that are decided dynamically using a variable during the program runtime based on the variable value.
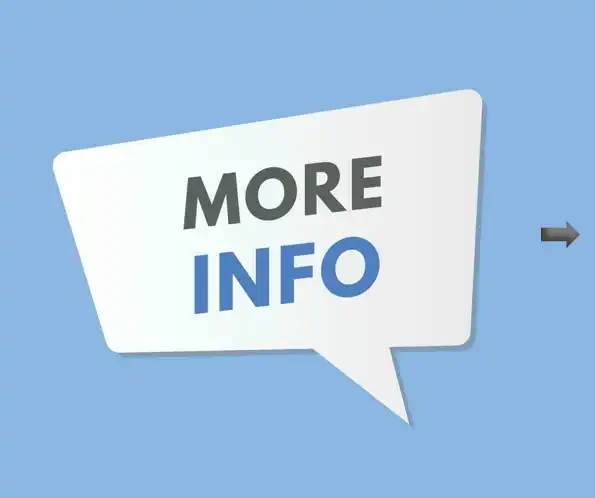