COBOL Table Subscript
Subscript is used to access the table data. It represents the number of table occurrences. It is a very straightforward value, and each occurrence can be accessed by using the occurrence number as a subscript.
Points to Note -
- OCCURS clause automatically creates a subscript value for each OCCURS. However, the subscript needs a separate variable declared in the program to refer to it.
- Subscript value is any positive number.
- The subscript value ranges from 1, and the maximum value limitation equals the number of OCCURS.
Diagram -
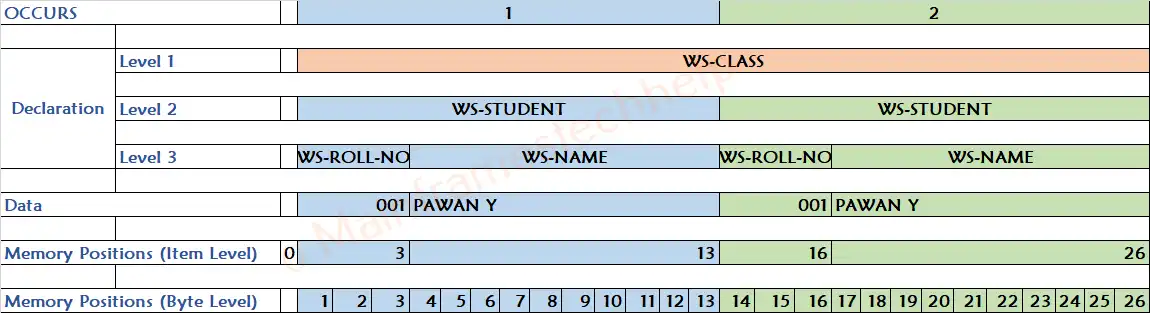
In the above diagram, the OCCURS represents subscript. Let us use the below declaration for a better understanding of subscript -
01 WS-CLASS.
03 WS-STUDENT OCCURS 2 TIMES.
05 WS-ROLL-NO PIC X(03).
05 WS-NAME PIC X(10).
WS-STUDENT has 2 OCCURRENCES. The subscript always starts at one and increases by one to refer to the next occurrence—the total number of occurrences accessed is WS-STUDENT (1) and WS-STUDENT (2).
How to declare a Subscript?
- The subscript should be declared in a WORKING-STORAGE SECTION.
- The subscript is declared as S9(04) COMP.
The subscript declaration is as follows -
01 WS-SUB PIC S9(04).
How to access table using subscript?
Syntax for accessing table items using subscript shown below -
table-data-item (subscript-variable)
The student details table can be accessed using subscripts for the above example is -
WS-STUDENT (WS-SUB).
How to initialize the Subscript?
MOVE statement initializes the subscript. The results are unpredictable if the subscript is used without initializing. The lowest possible subscript value is 1.
Initializing the above subscript as follows -
MOVE 1 TO WS-SUB.
How to increment/decrement the Subscript?
Subscript can increment by the ADD operator and decrement by the SUBTRACT operator. A COMPUTE statement can be used to do both.
Incrementing subscript from the above example as follows -
ADD 1 TO WS-SUB.
or
COMPUTE WS-SUB = WS-SUB + 1.
Decrementing subscript for the above example as follows -
SUBTRACT 1 FROM WS-SUB.
or
COMPUTE WS-SUB = WS-SUB - 1.
Possible Errors (SOC4) -
SOC4 error occurs in the following scenarios -
- Trying to access the table with the subscript value that is greater than the maximum number of table occurrences.
- Trying to access the table element with an uninitialized subscript.
Solution: -
Debug the program to verify the Subscript value during the abend. Check whether the subscript value exceeds the maximum value or is not initialized.
Practical Example -
Scenario - Declaring, initializing, incrementing an subscript and using it to navigate the table.
Code -
----+----1----+----2----+----3----+----4----+----5----+
...
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-CLASS.
03 WS-STUDENT OCCURS 2 TIMES.
05 WS-ROLL-NO PIC X(03).
05 WS-NAME PIC X(10).
* Declaring a subscript
01 WS-SUB PIC S9(04) COMP.
...
PROCEDURE DIVISION.
* Initializing the subscript to 1
MOVE 1 TO WS-SUB.
MOVE "001PAWAN Y" TO WS-STUDENT (WS-SUB).
* Incrementing subscript by 1
COMPUTE WS-SUB = WS-SUB + 1.
MOVE "002KUMAR" TO WS-STUDENT (WS-SUB).
* Displaying full table using subscript
PERFORM VARYING WS-SUB FROM 1 BY 1 UNTIL WS-SUB > 2
DISPLAY "STUDENT" WS-SUB " - " WS-STUDENT(WS-SUB)
END-PERFORM.
...
JCL to execute the above COBOL program −
//MATEPKRJ JOB MSGLEVEL=(1,1),NOTIFY=&SYSUID //* //STEP01 EXEC PGM=TBSUBSCR //STEPLIB DD DSN=MATEPK.COBOL.LOADLIB,DISP=SHR //SYSOUT DD SYSOUT=*
Output -
STUDENT0001 - 001PAWAN Y STUDENT0002 - 002KUMAR