Literal
The literal is the constant value that is assigned to the variable. Literals can represent various types of data, such as number (numeric data type), string (alphabetic or alphanumeric data type), or a figurative constant (for both).
Literals are two types –
- Non-numeric literals.
- Numeric literals.
Non-numeric literals -
Non-numeric literals are the alphabetic or alpha-numeric string enclosed between single (' ') or double (" ") quotation marks.. It can contain any allowed character from the character set (A-Z, a-z, 0-9, and special characters).
Syntax -
VALUE "non-numeric-literal"
VALUE 'non-numeric-literal'
VALUE non-numeric-figurative-constant
For example - Declaring a variable to store a value HELLO.
01 WS-VAR PIC A(05) VALUE "HELLO".
- non-numeric-literal-value - Specifies the non-numeric literal value. From the example - it is HELLO.
- non-numeric-figurative-constant - Specifies the non-numeric figurative constant. For example - SPACE, SPACES.
Guidelines -
Non-numeric literals follow the below guidelines -
- The quotation marks(" ") or apostrophes(' ') are excluded from the literal.
- Non-numeric literal minimum length is 1 character and maximum is 256 characters.
- Non-numeric literals are of type alphabetic or alpha-numeric.
Numeric literals -
A numeric literal is a numeric constant that is a combination of digits (0 through 9), a sign character (+ or -), and a decimal point(.). Quotation marks(" ") or apostrophes(' ') not required. Every numeric literal is of numeric data type.
Syntax -
VALUE numeric-literal.
VALUE numeric-figurative-constant.
For example - Declaring a variable of numeric type to store a value 1234.
01 WS-VAR PIC 9(04) VALUE 1234.
- numeric-literal - Specifies the numeric value. From the example, it is 1234.
- numeric-figurative-constant - Specifies the numeric figurative constant. For example, ZERO, ZEROES.
Guidelines -
Numeric literals follow the below rules -
- The minimum length is 1, and the maximum is 18 digits.
- It allows only one sign character. If the sign is coded as part of the literal, it should be the literal's first character.
- It allows only one decimal point. If a decimal point is part of the literal, it is considered an assumed decimal point.
Numeric Literal Types -
Numeric literals are again classified into two types, and those are -
- Fixed-point numbers.
- Floating-point numbers.
Fixed-point numeric literals -
Fixed-point numeric literals are numbers with a signed value (i.e., without mantissa and exponent). For example - +124567, 1234, -99382 etc,.
Floating-point numeric literals -
Floating-point numeric literals are specified in the form of mantissa and exponent. The below format specifies floating-point literal values -
[+/-] mantissa E [+/-] exponent
For example - +9.999E-3 (equal to 0.009999), where 9.999 is mantissa and 3 is exponent.
Practical Example -
Scenario - Example to describe how the literals declared in COBOL program.
Code -
----+----1----+----2----+----3----+----4----+----5----+
IDENTIFICATION DIVISION.
PROGRAM-ID. LITERAL.
AUTHOR. MTH.
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-VARIRABLES.
05 WS-NNUM-LIT PIC X(40)
VALUE "COBOL IS LEGACY PROGRAMMING LANGUAGE".
05 WS-NUM-LIT PIC 9(05) VALUE 256.
05 WS-FP-NUM-LIT PIC S9(05) VALUE -128.
05 WS-FLP-NUM-LIT PIC -99V9(3)E-99.
PROCEDURE DIVISION.
DISPLAY "NON-NUMERIC LITERAL: " WS-NNUM-LIT.
DISPLAY "NUMERIC LITERAL: " WS-NUM-LIT.
DISPLAY "FIXED-POINT NUMERIC LITERAL: " WS-FP-NUM-LIT.
MOVE -9.999E-3 TO WS-FLP-NUM-LIT.
DISPLAY "FLOATING-POINT NUMERIC LITERAL: " WS-FLP-NUM-LIT.
STOP RUN.
Output -
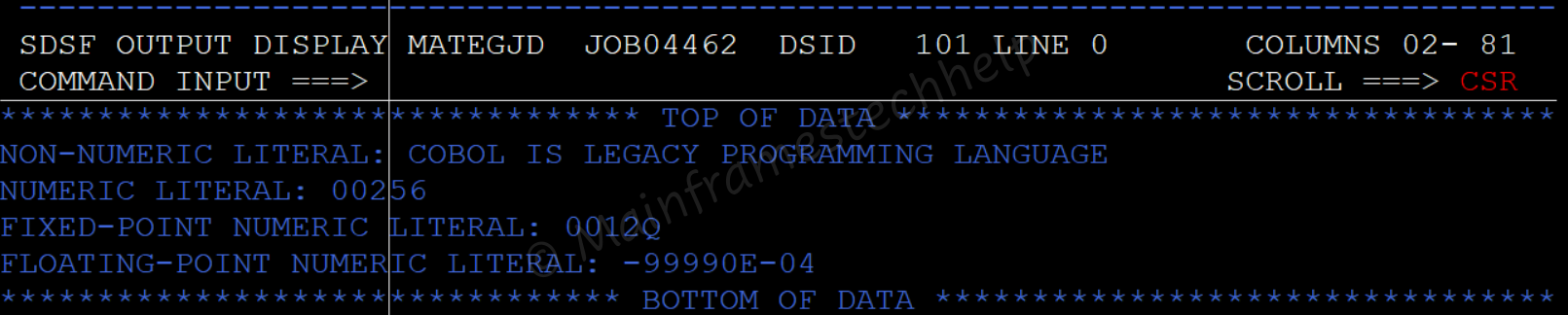
Explaining Example -
- In the above example, WS-NNUM-LIT is the non-numeric literal variable assigned with non-numeric literal COBOL IS LEGACY PROGRAMMING LANGUAGE.
- WS-NUM-LIT and WS-FP-NUM-LIT are fixed-point numeric variables assigned with literals 256 and -128. WS-FLP-NUM-LIT is a floating-point numeric literal variable assigned with -9.999E-3.
- The input value mantissa is 9.999(without decimal). However, the declaration is 99V9(3). So the mantissa changed to 99.990(without decimal), and the exponent decreased by 1. i.e., the exponent changed from -3 to -4. The final value displayed is -99990E-4.