COBOL Table Index
Indexes are used to access table data. An index refers to the table element as the number of displacement positions from the table starting position. Indexes are designed to be stored in the main memory to increase the accessing speed.
How the Index is declared?
Indexes are declared using the INDEXED BY phrase followed by the index name along with the OCCURS clause, as shown below -
01 WS-CLASS.
03 WS-STUDENT OCCURS 2 TIMES INDEXED BY WS-IDX.
05 WS-ROLL-NO PIC X(03).
05 WS-NAME PIC X(10).
Index variable doesn't require additional declaration in DATA DIVISION.
How to access the table using index?
Syntax for accessing table item using index shown below -
table-data-item (index-name)
The student details can be accessed using index for the above example is -
WS-STUDENT (WS-IDX)
Index value Calculation -
The index-value (starting position of data item) is calculated using the below formulae -
index-value = ((occurrence-number - 1) * occurrence-length) + 1
Therefore, for the second occurrence of WS-STUDENT, the binary value in WS-IDX is ((2 - 1) * 13) + 1, which is 14. So, when accessing WS-STUDENT (2), the data is retrieved starting at 14th byte + 13 bytes. Refer to the Diagram below for more understanding -
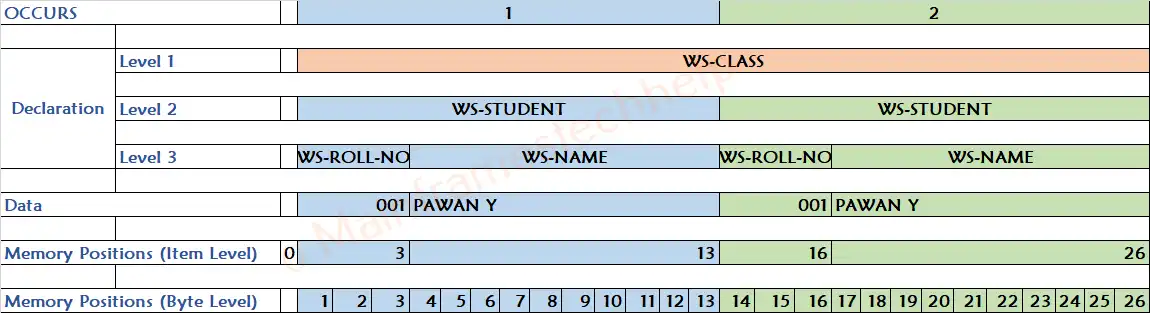
How to initialize the INDEX?
Index variable can’t be initialized during its declaration or using MOVE statement. SET statement is used to initialize the index variable. PERFORM VARYING statement can also initialize the index while processing the table in a loop. If the index is used with initializing it, the results are unpredictable. The lowest possible value for the index is 1. Initializing the index as follows -
SET WS-IDX TO 1.
How to increment/decrement the INDEX?
SET statement increases or decreases the index value. PERFORM or SEARCH statements can also increase or decrease the index.
Incrementing the index as follows –
SET WS-IDX UP BY 1.
Decrementing the index as follows –
SET WS-IDX DOWN BY 1.
Possible Errors (SOC4) -
SOC4 error occurs in the following scenarios -
- Trying to access the table with an index value that is greater than the maximum number of table occurrences.
- Trying to access the table element with an uninitialized index.
Solution: -
Debug the program to verify the index value at the time of abend. It is always good to check the index value for its range to avoid errors.
Practical Example -
Scenario - Declaring, initializing, incrementing an index and using it to navigate the table.
Code -
----+----1----+----2----+----3----+----4----+----5----+
...
DATA DIVISION.
WORKING-STORAGE SECTION.
* Declaring table with index
01 WS-CLASS.
03 WS-STUDENT OCCURS 2 TIMES INDEXED BY WS-IDX.
05 WS-ROLL-NO PIC X(03).
05 WS-NAME PIC X(10).
...
PROCEDURE DIVISION.
* Initializing index to 1
SET WS-IDX TO 1.
MOVE "001PAWAN Y" TO WS-STUDENT(WS-IDX).
* Incrementing index by 1
SET WS-IDX UP BY 1.
MOVE "002KUMAR" TO WS-STUDENT(WS-IDX).
* Displaying full table using index
PERFORM VARYING WS-IDX FROM 1 BY 1 UNTIL WS-IDX > 2
DISPLAY "STUDENT - " WS-STUDENT(WS-IDX)
END-PERFORM.
...
Output -
STUDENT - 001PAWAN Y STUDENT - 002KUMAR