COBOL Data Item (Variable) Declaration
Variable declaration syntax is an important aspect of COBOL programming to produce reliable and efficient code. Declaring variables is important and is required to process the data within the program.
All the variables should be declared in the DATA DIVISION under sections like WORKING-STORAGE SECTION or LINKAGE SECTION.
The variable declaration is as follows -
level-number data-item|variable-name
PIC data-type-character(length)
[VALUE literal-value] [additional-clauses].
Note! All statements coded in [ ] are optional.
For example - Declaring a variable of alphanumeric type to store a value "MAINFRAMES".
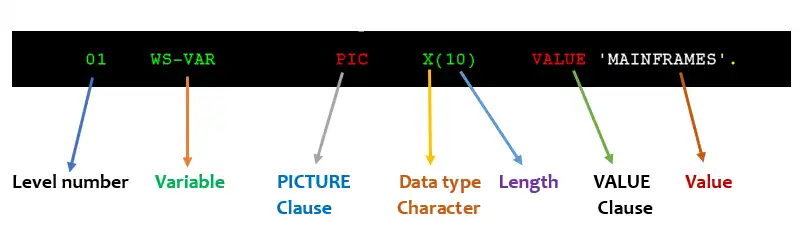
- level-number - Refers to the level number of the declaration from 01 to 49. From the example, it is 01.
- variable-name - Refers to the name of the variable. From the example, it is WS-VAR.
- data-type-character - Refers to the type of the variable. From the example, it is Alpha-numeric(X).
- variable-length - Refers to the variable length to store the data. From the example, it is 10.
- literal-value - Refers to the initial value assigned to the variable. From the example, it is MAINFRAMES.
We will discuss the below topics in the next immediate chapters -
- Level Number
- Data item | Variable
- PICTURE Clause
- Data Types
- USAGE Clause
- VALUE Clause
- Data Type Justifications
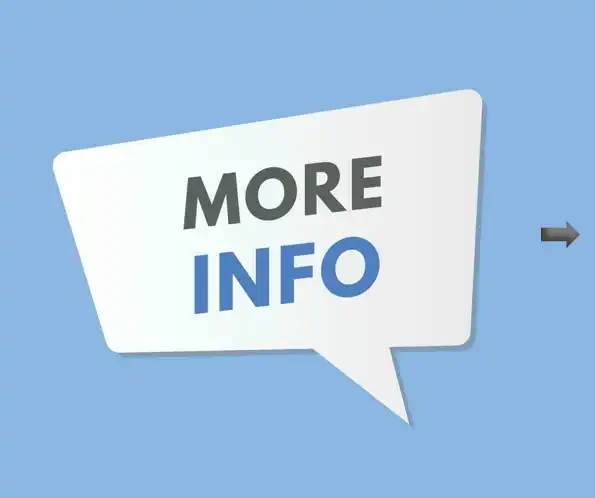